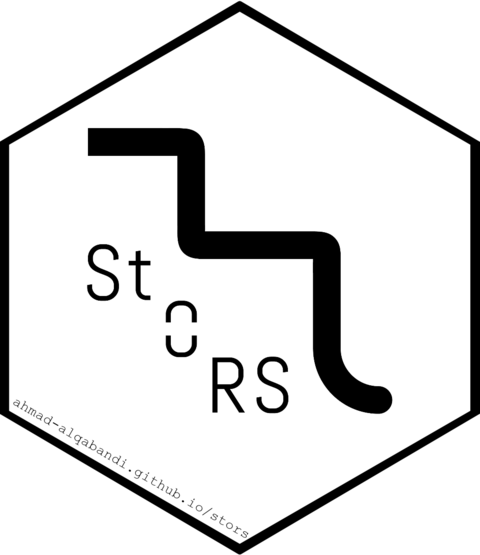
Sampling from Normal Distribution
srnorm.Rd
The srnorm()
function generates random samples from a Normal distribution using the STORS algorithm.
It employs an optimized proposal distribution around the mode and Adaptive Rejection Sampling (ARS) for the tails.
Value
A numeric vector of length n
containing samples from the Normal distribution with the specified
mean
and sd
.
NOTE: When the x
parameter is specified, it is updated in-place with the simulation for performance reasons.
Details
The Normal distribution has the probability density function (PDF): \(f(x | \mu, \sigma) = \frac{1}{\sigma\sqrt{2\pi}} \exp\left(-\frac{(x - \mu)^2}{2\sigma^2}\right),\) where:
- \(\mu\)
is the mean of the distribution, which determines the centre of the bell curve.
- \(\sigma\)
is the standard deviation, which controls the spread of the distribution (\(\sigma > 0\)).
These two functions are for sampling using the STORS algorithm based on the proposal that has been constructed using srnorm_optimize
.
By default, srnorm()
samples from a standard Normal distribution (mean = 0
, sd = 1
).
The proposal distribution is pre-optimized at package load time using srnorm_optimize()
with
steps = 4091
, creating a scalable proposal centred around the mode.
If srnorm()
is called with custom mean
or sd
parameters, the samples are generated
from the standard Normal distribution, then scaled and location shifted accordingly.
See also
srnorm_optimize
to optimize the custom or the scaled proposal.
Examples
# Generate 10 samples from the standard Normal distribution
samples <- srnorm(10)
print(samples)
#> [1] 0.4243311 1.4301971 -1.0563776 0.7653815 1.2819099 -0.5776533
#> [7] 0.6286107 1.0763716 -0.5866452 -0.7403679
# Generate 10 samples using a pre-allocated vector
x <- numeric(10)
srnorm(10, x = x)
#> [1] 1.0800536 -0.7451148 -0.7294962 -0.4985642 0.2222929 -0.2952098
#> [7] -1.8884170 0.0737074 0.8794445 1.0396361
print(x)
#> [1] 1.0800536 -0.7451148 -0.7294962 -0.4985642 0.2222929 -0.2952098
#> [7] -1.8884170 0.0737074 0.8794445 1.0396361
# Generate 10 samples from a Normal distribution with mean = 2 and sd = 3
samples <- srnorm(10, mean = 2, sd = 3)
print(samples)
#> [1] 3.86374215 6.52516141 -1.09233696 2.72138269 1.24684771 1.99993077
#> [7] 6.16478822 -0.05879545 2.11829241 1.87991590