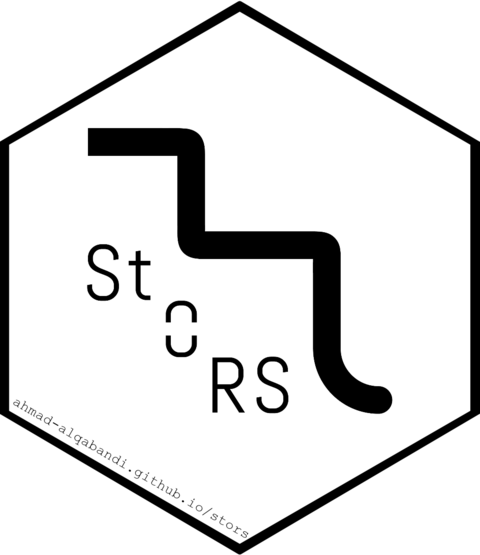
Print proposals
print_proposals.Rd
This function prints details of all proposals stored by the user. It provides information on each proposal, including the proposal name, size, efficiency, and other relevant details.
Examples
# First, let's create a proposal to sample from a standard normal distribution
f_normal <- function(x) { 0.3989423 * exp(-0.5 * x^2) }
normal_proposal = build_proposal(f = f_normal, modes = 0, lower = -Inf, upper = Inf, steps = 1000)
print(normal_proposal)
#>
#> ── Proposal Summary ────────────────────────────────────────────────────────────
#> • Total steps: 1,000
#> • Steps range: [-2.876766, 2.876766]
#> • Sampling efficiency: 99.56%
# `print_proposals()` prints all proposals stored in R's internal data directory.
# To see this, we first save `normal_proposal` using `save_proposal()`
save_proposal(normal_proposal, "normal")
# Since `normal_proposal` is now stored on this machine,
# we can confirm this by printing all saved proposals
print_proposals()
#>
#> ── Proposals Data ──────────────────────────────────────────────────────────────
#> Name | Size (KB) | Date
#> -----------------------------------------------
#> normal | 23.05 | 2025-03-10 14:27:27
#> -----------------------------------------------
#> Total Size: 23.05
# Example 2: Create and Save a proposal for a Bimodal Distribution
f_bimodal <- function(x) {
0.5 * (1 / sqrt(2 * pi)) * exp(-(x^2) / 2) +
0.5 * (1 / sqrt(2 * pi)) * exp(-((x - 4)^2) / 2)
}
modes_bimodal = c(0, 4)
bimodal_proposal = build_proposal(f = f_bimodal, modes = modes_bimodal,
lower = -Inf, upper = Inf, steps = 1000)
save_proposal(bimodal_proposal, "bimodal")
print(bimodal_proposal)
#>
#> ── Proposal Summary ────────────────────────────────────────────────────────────
#> • Total steps: 1,000
#> • Steps range: [-2.560912, 6.560912]
#> • Sampling efficiency: 99.42%
# To print all stored proposals after saving bimodal_proposal
print_proposals()
#>
#> ── Proposals Data ──────────────────────────────────────────────────────────────
#> Name | Size (KB) | Date
#> -----------------------------------------------
#> bimodal | 26.88 | 2025-03-10 14:27:27
#> normal | 23.05 | 2025-03-10 14:27:27
#> -----------------------------------------------
#> Total Size: 49.93